Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 | 31 |
Tags
- 치명적 귀여움
- 데이트
- A. Steed 2: Cruise Control
- codejam
- CodeJam 2017 Round 1B
- 냥이
- 발산
- 발산맛집
- 스코티쉬 스트레이트
- 소호정
- 카페
- RED CAT COFFEE X LOUNGE
- 커플
- 소호정본점
- 파버스
- coffee
- 파머스테이블
- 고양이
- 고양이는 언제나 귀엽다
- 안동국시
- CDJ
- 부모님과
- 먹기좋은곳
- 스파게티
- 냥스토리
- 냥냥
- 발산역 근처 카페
- 레스토랑
- 양재맛집
- 스테이크
Archives
- Today
- Total
hubring
Linear Regression의 cost 최소화 원리 본문
[참고] 모두를 위한 딥러닝 - 기본적인 머신러닝과 딥러닝 강좌
How to minimize cost?
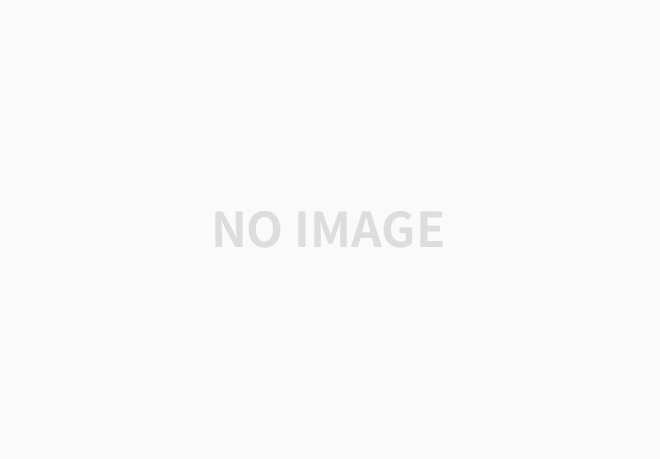
- 최소값을 찾아내야함.
Gradient descent algorithm
- 경사에 따라 내려가 최소값을 찾는 알고리즘.
- minimize cost function
- 최소값을 찾는 많은 문제에 사용됨.
How it works?
- 어떤 점에서 시작 W, b 값을 조금씩 변경하며 최소값을 찾음.
Formal definition
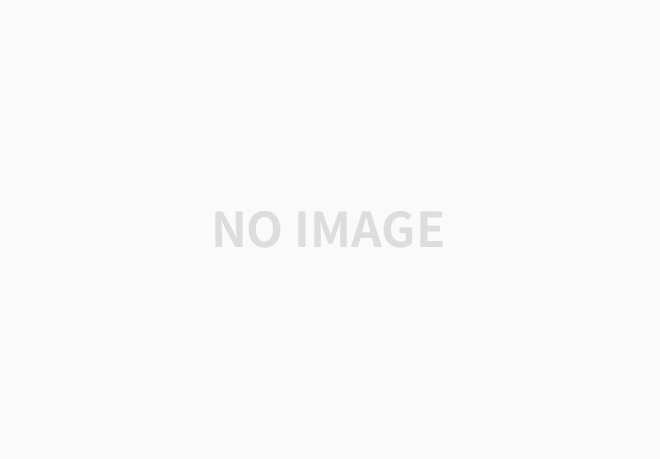
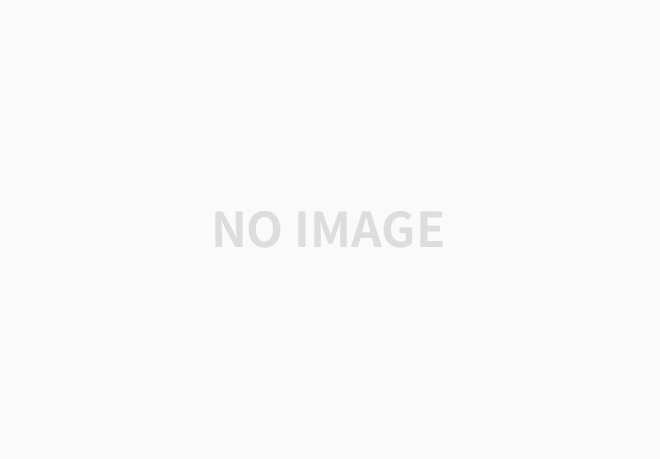
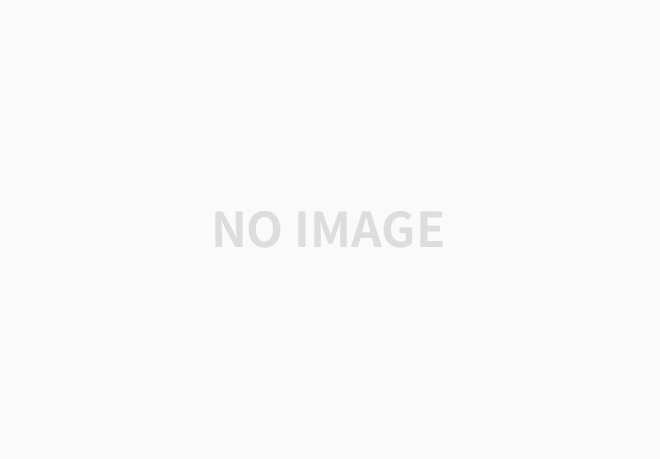
- 미분하여 W 값을 구함.
Convex function
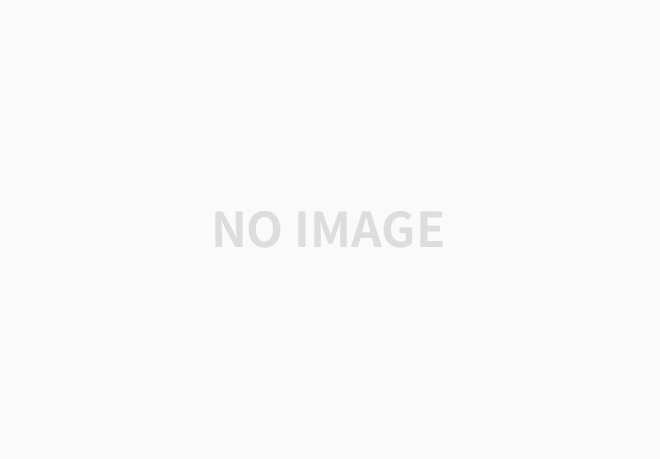
- 어느 지점에서 시작하든 최소 값을 찾는 방향으로 갈 수 있음.
- cost 함수가 위와 같은 모양일 경우 Grdient descent algorithm을 사용할 수 있다.
직접 cost 최소화 알고리즘 적용해보기
W의 변화에 따른 오차값 그래프 확인
import numpy as np
import tensorflow.compat.v1 as tf
import matplotlib.pyplot as plt
tf.disable_v2_behavior()
X = [1, 2, 3]
Y = [1, 2, 3]
W = tf.placeholder(tf.float32)
hypotheis = X * W
cost = tf.reduce_mean(tf.square(hypotheis - Y))
sess = tf.Session()
sess.run(tf.global_variables_initializer())
W_val = []
cost_val = []
for i in range(-30, 50) :
feed_W = i * 0.1
curr_cost, curr_W = sess.run([cost, W], feed_dict={W: feed_W})
W_val.append(curr_W)
cost_val.append(curr_cost)
plt.plot(W_val, cost_val)
plt.show()
미분을 통해 기울기 값이 최소값이 되도록 업데이트
GradientDescent
learning_rate = 0.1
gredient = tf.reduce_mean((W * X - Y) * X)
descent = W - learning_rate * gredient
update = W.assign(descent)
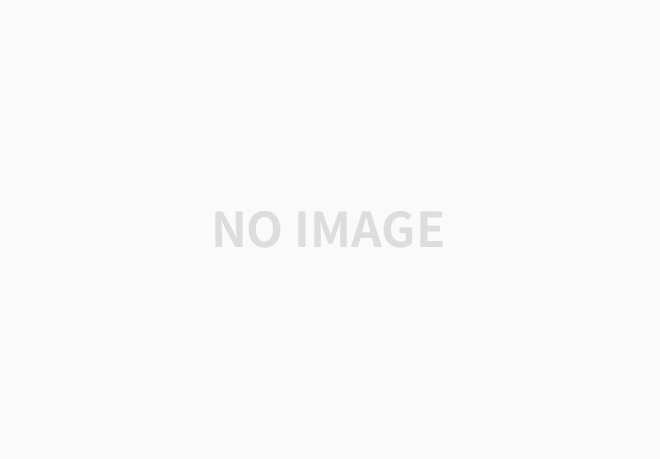
전체 코드
import numpy as np
import tensorflow.compat.v1 as tf
import matplotlib.pyplot as plt
tf.disable_v2_behavior()
x_data = [ 1, 2, 3]
y_data = [ 1, 2, 3]
X = tf.placeholder(tf.float32)
Y = tf.placeholder(tf.float32)
W = tf.Variable(tf.random_normal([1]), tf.float32)
hypotheis = X * W
cost = tf.reduce_mean(tf.square(hypotheis - Y))
# minimize
learning_rate = 0.1
gredient = tf.reduce_mean((W * X - Y) * X)
descent = W - learning_rate * gredient
update = W.assign(descent)
sess = tf.Session()
sess.run(tf.global_variables_initializer())
W_val = []
cost_val = []
for step in range(21) :
sess.run(update, feed_dict={ X : x_data, Y : y_data})
print(step, sess.run(cost, feed_dict={ X : x_data, Y : y_data}), sess.run(W))
'AI > Tensorflow' 카테고리의 다른 글
TensorFlow로 파일에서 데이터 읽어오기 (0) | 2021.03.05 |
---|---|
Multi-variable linear regression (0) | 2021.02.24 |
Linear Regression (0) | 2021.02.23 |
머신 러닝 기본 (0) | 2021.02.23 |
k-인접이웃 분류모형 (0) | 2019.09.18 |